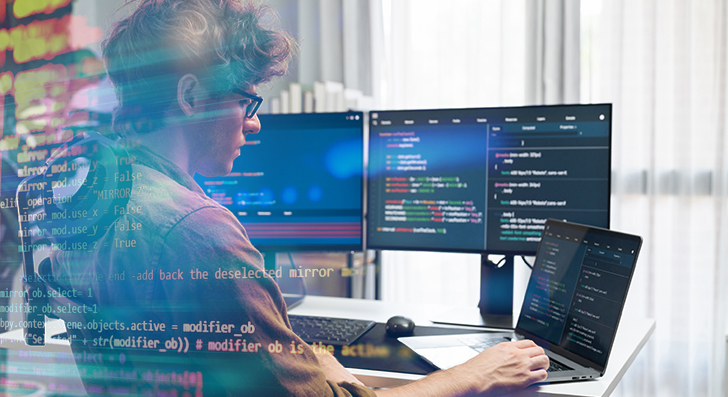
Scalability suggests your software can tackle expansion—a lot more customers, extra facts, and a lot more site visitors—with out breaking. As a developer, making with scalability in mind will save time and anxiety later. Below’s a clear and realistic guidebook that will help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of your system from the beginning. Lots of programs are unsuccessful after they mature quickly because the initial structure can’t manage the additional load. As being a developer, you'll want to think early about how your procedure will behave under pressure.
Start off by designing your architecture for being adaptable. Avoid monolithic codebases the place everything is tightly connected. Alternatively, use modular design or microservices. These patterns split your application into lesser, independent elements. Each individual module or service can scale on its own with no influencing The complete method.
Also, think of your databases from day one particular. Will it need to manage one million customers or perhaps 100? Pick the correct variety—relational or NoSQL—according to how your knowledge will mature. Plan for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential stage is in order to avoid hardcoding assumptions. Don’t create code that only functions below existing problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or party-pushed units. These help your application manage a lot more requests with out obtaining overloaded.
Whenever you build with scalability in your mind, you are not just planning for achievement—you're lowering potential headaches. A well-prepared technique is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the best Database
Choosing the ideal databases is really a key Element of making scalable programs. Not all databases are built exactly the same, and utilizing the Mistaken one can gradual you down as well as trigger failures as your application grows.
Commence by comprehending your data. Can it be remarkably structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great match. These are solid with relationships, transactions, and regularity. They also support scaling approaches like study replicas, indexing, and partitioning to take care of a lot more targeted visitors and info.
If your knowledge is a lot more versatile—like person action logs, product catalogs, or paperwork—consider a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured information and might scale horizontally extra very easily.
Also, think about your read through and write patterns. Will you be doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently managing a heavy compose load? Look into databases that could cope with high compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also sensible to Assume in advance. You may not require Superior scaling characteristics now, but picking a databases that supports them usually means you received’t need to switch later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details depending on your access styles. And always monitor database performance as you increase.
In a nutshell, the best databases is dependent upon your app’s construction, speed requirements, and how you count on it to develop. Consider time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each little hold off provides up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Begin by writing clean up, basic code. Stay clear of repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular functions. Keep the features short, concentrated, and straightforward to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well extensive to run or utilizes far too much memory.
Following, look at your databases queries. These usually gradual items down more than the code by itself. Make sure Every single query only asks for the information you truly get more info want. Stay clear of Pick *, which fetches every thing, and in its place pick precise fields. Use indexes to speed up lookups. And keep away from executing too many joins, In particular throughout huge tables.
For those who discover the exact same details becoming asked for many times, use caching. Shop the results temporarily working with tools like Redis or Memcached which means you don’t need to repeat high priced functions.
Also, batch your database operations when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app far more successful.
Make sure to test with big datasets. Code and queries that perform high-quality with a hundred documents may well crash if they have to take care of one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These methods support your software keep smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server performing all the perform, the load balancer routes customers to different servers dependant on availability. This suggests no solitary server will get overloaded. If just one server goes down, the load balancer can ship traffic to the others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this easy to build.
Caching is about storing data quickly so it may be reused quickly. When buyers request exactly the same information and facts once again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may provide it in the cache.
There's two frequent types of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching minimizes databases load, improves pace, and makes your app extra effective.
Use caching for things which don’t alter generally. And always be sure your cache is updated when info does improve.
In brief, load balancing and caching are easy but strong applications. With each other, they assist your app manage additional customers, remain rapid, and recover from difficulties. If you propose to mature, you will need both equally.
Use Cloud and Container Applications
To develop scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to rent servers and providers as you may need them. You don’t should invest in components or guess upcoming capacity. When traffic increases, you'll be able to include a lot more assets with only a few clicks or routinely employing automobile-scaling. When targeted traffic drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability instruments. You may target constructing your app rather than handling infrastructure.
Containers are another vital Software. A container deals your app and every thing it needs to operate—code, libraries, options—into just one unit. This makes it easy to maneuver your application in between environments, from your notebook on the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If a single part of your respective app crashes, it restarts it quickly.
Containers also help it become easy to different areas of your application into companies. You are able to update or scale pieces independently, that's perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments indicates you are able to scale rapid, deploy very easily, and Get better rapidly when challenges take place. If you prefer your app to increase without boundaries, get started making use of these instruments early. They save time, lessen hazard, and enable you to keep focused on creating, not repairing.
Keep track of Anything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is executing, place challenges early, and make better choices as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring basic metrics like CPU usage, memory, disk Room, and response time. These inform you how your servers and expert services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for users to load pages, how often errors happen, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified immediately. This helps you take care of difficulties rapid, typically just before customers even notice.
Checking is likewise valuable once you make alterations. Should you deploy a brand new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to serious problems.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct applications in position, you stay on top of things.
In short, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By planning carefully, optimizing properly, and utilizing the right equipment, you can Construct applications that grow effortlessly with out breaking stressed. Get started little, Assume large, and Create good.